Fuck LeetCode > Linked List > 203. Remove Linked List Elements > Solved in Java, Python, C++, JavaScript, C#, Go, Ruby > Repost or Contribute
LeetCode link: 203. Remove Linked List Elements, difficulty: Easy.
Given the head
of a linked list and an integer val
, remove all the nodes of the linked list that has Node.val == val
, and return the new head.
Example 1:
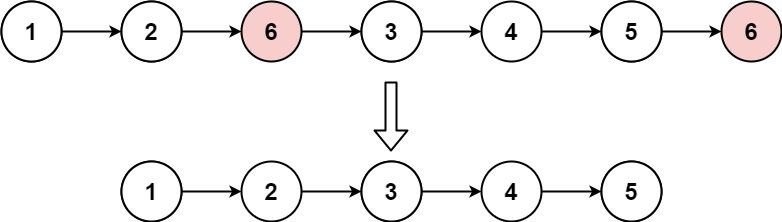
Input: head = [1,2,6,3,4,5,6], val = 6
Output: [1,2,3,4,5]
Example 2:
Input: head = [], val = 1
Output: []
Example 3:
Input: head = [7,7,7,7], val = 7
Output: []
Constraints:
- The number of nodes in the list is in the range
[0, 10000]
. 1 <= Node.val <= 50
0 <= val <= 50
Intuition
Assume that the node to be deleted in the linked list is
d
, and the previous node ofd
isp
, sop.next
isd
.To delete
d
, just setp.next = p.next.next
.Because
p.next.next
is used, the loop condition should bewhile (p.next != null)
instead ofwhile (p != null)
.But there is no node before the
head
node, which means that thehead
node needs to be treated specially.Is there a way to make the
head
node no longer special? In this way, there is no need to treat thehead
specially.Click to view the answer
The way is to introduce a
dummy
node,dummy.next = head
.
Complexity
Time complexity
O(N)
Space complexity
O(1)
Java #
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode removeElements(ListNode head, int val) {
var dummyHead = new ListNode();
dummyHead.next = head;
var node = dummyHead;
while (node.next != null) {
if (node.next.val == val) {
node.next = node.next.next;
} else {
node = node.next;
}
}
return dummyHead.next;
}
}
Python #
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def removeElements(self, head: Optional[ListNode], val: int) -> Optional[ListNode]:
dummy_head = ListNode()
dummy_head.next = head
node = dummy_head
while node.next:
if node.next.val == val:
node.next = node.next.next
else:
node = node.next
return dummy_head.next
C++ #
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* removeElements(ListNode* head, int val) {
auto dummyHead = new ListNode();
dummyHead->next = head;
auto node = dummyHead;
while (node->next != nullptr) {
if (node->next->val == val) {
auto next_node = node->next;
node->next = node->next->next;
delete next_node;
} else {
node = node->next;
}
}
return dummyHead->next;
}
};
JavaScript #
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
var removeElements = function (head, val) {
const dummyHead = new ListNode()
dummyHead.next = head
let node = dummyHead
while (node.next != null) {
if (node.next.val == val) {
node.next = node.next.next
} else {
node = node.next
}
}
return dummyHead.next
};
C# #
/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
public class Solution {
public ListNode RemoveElements(ListNode head, int val) {
var dummyHead = new ListNode();
dummyHead.next = head;
var node = dummyHead;
while (node.next != null) {
if (node.next.val == val) {
node.next = node.next.next;
} else {
node = node.next;
}
}
return dummyHead.next;
}
}
Go #
/**
* Definition for singly-linked list.
* type ListNode struct {
* Val int
* Next *ListNode
* }
*/
func removeElements(head *ListNode, val int) *ListNode {
dummyHead := &ListNode{}
dummyHead.Next = head
node := dummyHead
for node.Next != nil {
if node.Next.Val == val {
node.Next = node.Next.Next
} else {
node = node.Next
}
}
return dummyHead.Next
}
Ruby #
# Definition for singly-linked list.
# class ListNode
# attr_accessor :val, :next
# def initialize(val = 0, _next = nil)
# @val = val
# @next = _next
# end
# end
def remove_elements(head, val)
dummy_head = ListNode.new
dummy_head.next = head
node = dummy_head
until node.next.nil?
if node.next.val == val
node.next = node.next.next
else
node = node.next
end
end
dummy_head.next
end